Generating and Editing images
See Live Demo built using these code snippets
- Typescript
- Python
import { GoogleGenerativeAI } from "@google/generative-ai";
const genAI = new GoogleGenerativeAI(process.env.GEMINI_API_KEY);
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp",
generationConfig: {
responseModalities: ["text", "image"],
},
});
const prompt = 'Hi, can you create a 3d rendered image of a pig ' +
'with wings and a top hat flying over a happy ' +
'futuristic scifi city with lots of greenery?';
const result = await model.generateContent({
contents: [{ role: "user", parts: [{ text: prompt }] }],
});
result.response.candidates[0].content.parts.forEach(part => {
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
const img = document.createElement('img');
img.src = `data:${part.inlineData.mimeType};base64,${part.inlineData.data}`;
document.body.appendChild(img);
}
});
from google import genai
from google.genai import types
from PIL import Image
from io import BytesIO
client = genai.Client()
contents = ('Hi, can you create a 3d rendered image of a pig '
'with wings and a top hat flying over a happy '
'futuristic scifi city with lots of greenery?')
response = client.models.generate_content(
model="models/gemini-2.0-flash-exp",
contents=contents,
config=types.GenerateContentConfig(response_modalities=['Text', 'Image'])
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
image = Image.open(BytesIO(part.inline_data.data))
image.show()
Edit an image
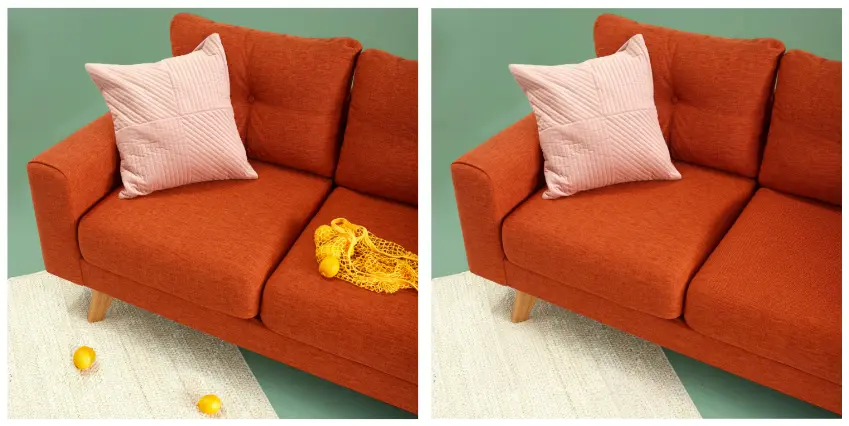
- Typescript
- Python
const genAI = new GoogleGenerativeAI(apiKey);
const model = genAI.getGenerativeModel({
model: "gemini-2.0-flash-exp",
generationConfig: {
responseModalities: ["text", "image"],
},
});
const base64Data = result.split(',')[1];
const byteCharacters = atob(base64Data);
const byteNumbers = new Array(byteCharacters.length);
for (let i = 0; i < byteCharacters.length; i++) {
byteNumbers[i] = byteCharacters.charCodeAt(i);
}
const byteArray = new Uint8Array(byteNumbers);
const blob = new Blob([byteArray], { type: result.split(';')[0].split(':')[1] });
const buffer = await blob.arrayBuffer();
const base64 = btoa(
new Uint8Array(buffer).reduce((data, byte) => data + String.fromCharCode(byte), '')
);
const response = await model.generateContent([
"Remove the lemons from the image",
{
inlineData: {
mimeType: blob.type,
data: base64
}
}
]);
response.response.candidates[0].content.parts.forEach(part => {
if (part.text) {
console.log(part.text);
} else if (part.inlineData) {
setResult(`data:${part.inlineData.mimeType};base64,${part.inlineData.data}`);
}
});
from google import genai
from PIL import Image
# Initialize the model
model = genai.GenerativeModel("gemini-2.0-flash-exp")
def edit_image(image_path, edit_prompt):
image = Image.open(image_path)
response = model.generate_content(
[edit_prompt, image],
config=genai.types.GenerateContentConfig(
response_modalities=['Text', 'Image']
)
)
for part in response.candidates[0].content.parts:
if part.text is not None:
print(part.text)
elif part.inline_data is not None:
# Display or save the edited image
edited_image = Image.open(BytesIO(part.inline_data.data))
edited_image.show()